Learn how to create LP object Python and understand its applications with this detailed beginner-friendly guide. Perfect for developers!
Introduction
When working with optimization problems, Python offers a powerful ecosystem of libraries to make the process efficient and straightforward. One of these libraries is PuLP, which allows users to solve Linear Programming (LP) problems effectively. If you’re wondering how to create LP object Python, this blog post will walk you through the concept, step-by-step instructions, and practical examples. Let’s dive in to create LP object Python!
What Is an LP Object?
Linear Programming (LP) is a mathematical method for optimizing an objective function, subject to a set of constraints. To create LP object Python, an LP object represents this optimization problem. With libraries like PuLP, you can define, solve, and interpret LP problems seamlessly.
An LP object allows you to:
- Define an objective function (e.g., maximize profit or minimize cost).
- Add constraints to ensure the solution is feasible.
- Solve the problem efficiently and retrieve the optimal values.
For instance, you can use an LP object to minimize costs when you create LP object Python, maximize profits, or allocate resources efficiently in a business scenario. Whether you’re managing inventory, optimizing routes, or scheduling employees, LP provides powerful tools to simplify these complex problems and to create LP object Python.
Why Use Python for LP?
Python’s versatility and its vast array of libraries make it ideal for Linear Programming. Here are some advantages:
- Ease of Use: Libraries like PuLP have intuitive syntax that simplifies complex operations. Unlike other programming languages, Python allows you to implement LP solutions with minimal code.
- Integration: Python integrates well with other tools, making it easier to analyze and visualize results. You can use libraries like matplotlib or pandas for reporting and charting your results.
- Community Support: Python’s active community ensures that you’ll always find resources, tutorials, and support when needed. Beginners and experts alike can benefit from this vast knowledge base.
Moreover, create LP object Python is free and open-source, making it accessible to anyone interested in learning Linear Programming.
Getting Started: Setting Up Your Environment
Before you create LP object Python, you need to install the required libraries. The most commonly used library for this purpose is PuLP.
Step 1: Install PuLP
To get started, install the PuLP library using the following command:
pip install pulp
Latest version of PuLP will be installed on your system. Make sure you have pip set up properly before running the command.
Step 2: Verify Installation
After installation, verify that PuLP is correctly installed by running the following script in your Python environment:
import pulp
print(pulp.__version__)
If the version number is displayed, you are ready to proceed. If not, double-check your installation steps or consult the official PuLP documentation.
How to Create an LP Object in Python
Now that your environment is ready, let’s move on to the main objective: how to create LP object Python. Below is a detailed walkthrough of the process, broken into simple steps.
Step 1: Import PuLP
Before you begin, import the necessary modules from the PuLP library. These modules will help you define problems, variables, and constraints:
from pulp import LpProblem, LpMaximize, LpMinimize, LpVariable
Step 2: Define the Problem
The first step in any LP problem is defining whether you want to maximize or minimize your objective function. This is done by creating an LpProblem object:
# Define the problem
task = LpProblem(“Simple LP Problem”, LpMaximize)
In this example, we’ve named the problem “Simple LP Problem” and specified that it’s a maximization task. If you were solving a minimization problem, you would use LpMinimize instead.
Also Read: Smartphone Repair Temecula
Step 3: Define Variables
Next, define the variables that will be used in the objective function and constraints. Variables typically represent quantities like the number of items to produce or allocate.
x = LpVariable(“x”, lowBound=0) # Variable x, lower bound 0
y = LpVariable(“y”, lowBound=0) # Variable y, lower bound 0
In this case, x and y represent two decision variables with a lower bound of zero (non-negative).
Step 4: Define the Objective Function
The objective function is the equation you aim to maximize or minimize. Here’s how you define it in PuLP:
task += 3 * x + 2 * y, “Objective Function”
This represents the equation 3x + 2y, where the coefficients indicate the contribution of each variable to the objective.
Step 5: Add Constraints
Constraints are rules that the solution must adhere to. They limit the values that variables can take. For example:
task += 2 * x + y <= 20, “Constraint 1”
task += 4 * x + 3 * y <= 40, “Constraint 2”
These constraints ensure that the solution is feasible within the given boundaries.
Step 6: Solve the Problem
Once the problem is defined, solving it is straightforward. Use the solve method:
task.solve()
# Output the status of the solution
print(“Status:”, pulp.LpStatus[task.status])
# Output the values of the variables
print(“x =”, x.varValue)
print(“y =”, y.varValue)
# Output the optimized objective function value
print(“Objective Value =”, pulp.value(task.objective))
This will display the solution status, the values of the variables, and the optimized value of the objective function. And you can create LP object Python
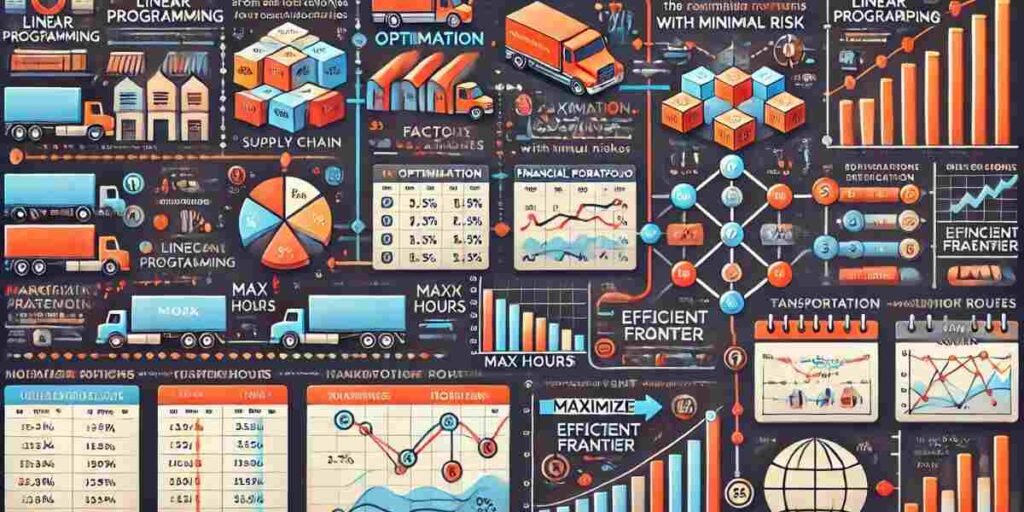
Real-World Applications of LP in Python
Linear Programming is widely used in various industries to solve complex problems efficiently and to create LP object Python. Here are some examples with detailed explanations:
Supply Chain Optimization
Supply chains involve multiple steps, including sourcing raw materials, manufacturing products, and distributing them to customers. Each of these steps incurs costs. By using LP, businesses can minimize these costs while ensuring timely delivery. For instance:
- Warehouse Optimization: Determine the optimal number of warehouses and their locations to minimize transportation costs.
- Transportation Planning: Decide the most cost-effective way to transport goods between factories and retailers.
- Inventory Management: Optimize stock levels to avoid overproduction or shortages.
A well-optimized supply chain not only reduces expenses but also improves customer satisfaction by ensuring product availability.
Financial Portfolio Optimization
Investors aim to maximize returns while minimizing risks. LP provides a systematic way to achieve this by helping select the best mix of investments. Key factors to create LP object Python include:
- Risk Tolerance: Ensure that the portfolio’s risk level aligns with the investor’s preferences.
- Diversification: Allocate investments across multiple asset classes to reduce risk.
- Constraints: Adhere to specific conditions, such as minimum or maximum investment amounts in certain sectors.
By applying LP, investors can make data-driven decisions that align with their financial goals.
Workforce Scheduling
Scheduling employees efficiently can be a daunting task, especially in industries like healthcare or retail. LP helps businesses:
- Allocate Shifts: Ensure all shifts are covered while minimizing overtime costs.
- Meet Employee Preferences: Incorporate employee preferences and availability into the schedule.
- Adhere to Regulations: Comply with labor laws regarding working hours and rest periods.
For example, a hospital can use LP to ensure there are enough doctors and nurses on each shift while minimizing costs and maintaining compliance with healthcare regulations.
Transportation Problems
Transportation problems involve moving goods from multiple sources (e.g., factories) to multiple destinations (e.g., retail stores) at the lowest cost. LP can solve these problems by:
- Minimizing Costs: Determine the cheapest routes while considering fuel, labor, and toll costs.
- Balancing Supply and Demand: Ensure that supply at each source meets demand at each destination.
- Improving Delivery Times: Optimize routes to reduce transit times and improve customer satisfaction.
For instance, a logistics company can use LP to plan routes for its delivery trucks, ensuring timely delivery at the lowest possible cost.
Exploring the Mathematical Foundation of Linear Programming
Linear Programming is based on a rich mathematical framework. LP involves optimizing a linear objective function subject to a set of linear constraints. These constraints form a feasible region, typically represented as a convex polyhedron. The optimal solution lies at one of the vertices of this polyhedron.
- Objective Function: This is the mathematical expression to optimize (e.g., maximize profit).
- Constraints: These limit the values of decision variables, creating boundaries.
- Feasible Region: The set of all points that satisfy the constraints.
Understanding these mathematical components enhances your ability to construct accurate and effective LP models.
Common Challenges When you Create LP object Python
To create LP object Python can sometimes be challenging. Here are common issues while you can create LP object Python and how to address them:
- Poorly Defined Objective Function: Ensure the coefficients and variables in your objective function accurately reflect your goals.
- Unrealistic Constraints: Double-check that constraints are practical and do not contradict each other.
- Variable Bounds: Missing bounds for variables can result in solutions that are infeasible or meaningless.
- Solver Errors: Ensure you have installed and configured the solver (e.g., CBC) correctly.
By being aware of these challenges, you can build robust LP models that solve problems effectively and you can create LP object Python.
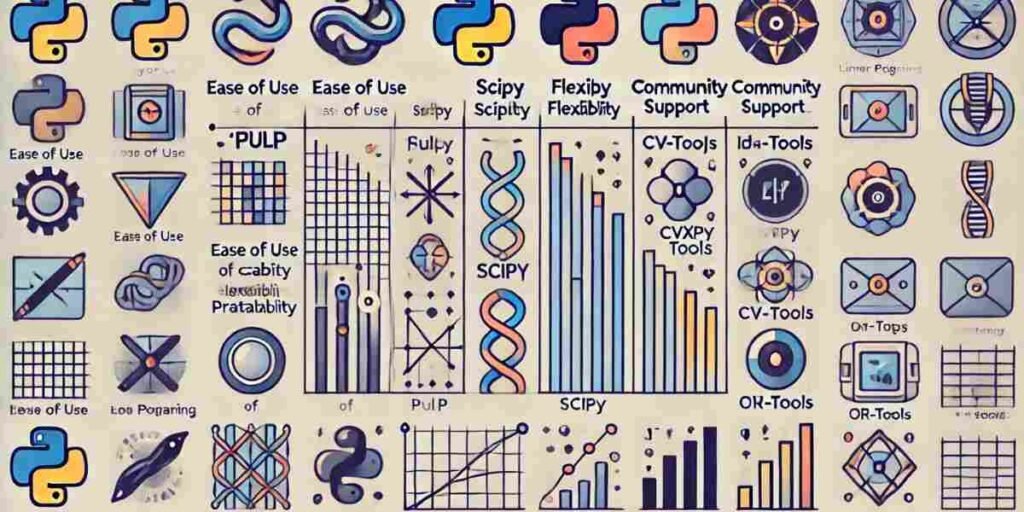
Comparing Python Libraries for Linear Programming
Python offers several libraries for Linear Programming, each designed to address different levels of complexity and specific use cases. Here’s a detailed comparison of some popular libraries to create LP object Python:
- PuLP: This is one of the most popular libraries for Linear Programming due to its simplicity and beginner-friendly interface. It provides built-in solvers and an easy syntax to define objective functions and constraints. PuLP is ideal for small to medium-sized problems and is great for learning and prototyping.
- SciPy: The optimize module in SciPy offers tools for solving optimization problems, including Linear Programming. However, it requires a bit more manual setup compared to PuLP, such as explicitly defining bounds and constraints in array format. SciPy is suitable for users who need flexibility and are comfortable working with arrays and numerical methods.
- CVXPY: This library is designed for convex optimization problems and supports complex constraints like integer programming. It is particularly powerful when dealing with problems that require advanced mathematical formulations. CVXPY is a great choice for academic research or high-level projects that involve intricate constraints and custom solvers.
- OR-Tools: Developed by Google, OR-Tools is a robust library for solving advanced optimization problems, including routing and scheduling. It is highly efficient for large-scale problems and integrates well with other Google tools. OR-Tools is ideal for businesses that need scalable solutions for logistics, transportation, or supply chain optimization.
Each library has its strengths and limitations. Beginners may find PuLP to be the easiest to start with, while advanced users might prefer the flexibility and scalability of CVXPY or OR-Tools. Understanding your project requirements, such as the size of the problem and the type of constraints involved, will help you choose the right library.
Advanced Features of PuLP for Complex LP Problems
PuLP is not limited to basic LP problems. Some advanced features to create LP object Python include:
- Integer Programming: Define variables as integers for problems like job scheduling.
- Custom Solvers: Use solvers like Gurobi or CPLEX for faster computation.
- Large-Scale Problems: Handle models with thousands of variables and constraints efficiently.
Leveraging these features can help solve more sophisticated optimization problems.
Future Trends in Optimization and Python
Optimization is evolving rapidly, and Python remains at the forefront. Future trends include:
- AI-Driven Optimization: Combining Linear Programming with machine learning for predictive modeling.
- Real-Time Solvers: Faster algorithms that can process dynamic data streams.
- Cloud-Based Solutions: Leveraging cloud computing for scalability and distributed problem solving.
Staying updated on these trends will ensure you remain competitive in the field of optimization.
FAQs to Create LP Object Python
1. What is an LP object in Python?
An LP object represents a Linear Programming problem, including its objective function and constraints. Python libraries like PuLP allow you to create and solve these objects efficiently.
2. Can I solve both maximization and minimization problems using Python?
Yes, Python supports both types. You can specify the problem type (maximize or minimize) when creating the LpProblem object.
3. Are there alternatives to PuLP for Linear Programming?
Yes, other libraries include SciPy, OR-Tools, and CVXPY. However, PuLP is one of the most user-friendly options for beginners.
4. What are some common errors when you create LP object Python?
- Forgetting to install the PuLP library.
- Not specifying bounds for variables, which can lead to unexpected results.
- Incorrectly defining constraints or the objective function.
5. Can I visualize LP solutions in Python?
Yes, libraries like matplotlib can be used to create graphs and visualize the solution space or results.
Conclusion
Creating an LP object in Python is a straightforward process, especially with libraries like PuLP. By following the steps outlined in this guide, you can define and solve optimization problems efficiently. Whether you’re a beginner or an experienced developer, understanding how to create LP object Python will open doors to solving complex real-world problems with ease.
Start exploring Linear Programming in Python today, and take your problem-solving skills to the next level!